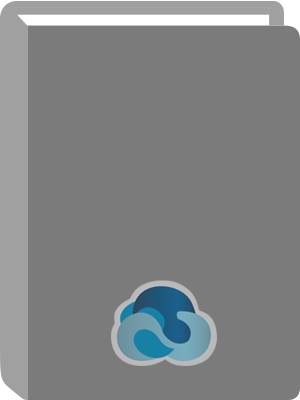
Title:
Algorithms and data structures : an approach in C
Personal Author:
Publication Information:
New York : Oxford Univ. Press, 1994
ISBN:
9780195114430
Available:*
Library | Item Barcode | Call Number | Material Type | Item Category 1 | Status |
---|---|---|---|---|---|
Searching... | 30000004967042 | QA76.73.C15 B68 1994 | Open Access Book | Book | Searching... |
Searching... | 30000004967034 | QA76.73.C15 B68 1994 | Open Access Book | Book | Searching... |
On Order
Summary
Summary
With numerous practical, real-world algorithms presented in the C programming language, Bowman's Algorithms and Data Structures: An Approach in C is the algorithms text for courses that take a modern approach. For the one- or two-semester undergraduate course in data structures, it instructs students on the science of developing and analyzing algorithms. Bowman focuses on both the theoretical and practical aspects of algorithm development. He discusses problem-solving techniques and introduces the concepts of data abstraction and algorithm efficiency. More importantly, the text does not present algorithms in a "shopping-list" format. Rather it provides actual insight into the design process itself.
Author Notes
Charles F.Bowman.
Table of Contents
1 Introduction |
1.1 Overview |
1.2 Why Study Algorithms? |
1.3 Why C? |
1.4 Coding Style |
1.5 What You Need to Know |
2 Algorithm Design |
2.1 How to Design an Algorithm |
2.2 Example 1: Fibonacci Numbers |
2.3 Example 2: Matrix Addition |
3 Static Data Structures |
3.1 Overview |
3.2 Arrays |
3.3 Ordered Lists |
3.4 Stacks |
3.5 Example Calculator |
3.6 Queues |
4 Recursion |
4.1 Introduction |
4.2 Factorial Numbers |
4.3 Writing Recursive Functions |
4.4 Use of Recursion |
5 Dynamic Data Structures |
5.1 Introduction |
5.2 Linked Lists |
5.3 Linked Lists Using Pointers |
5.4 List Processing |
5.5 Stacks Revisited |
5.6 Queues Revisited |
5.7 Dynamic Memory Allocation |
5.8 Simulation Example |
5.9 Doubly Linked Lists |
5.10 Generalized Lists |
6 Trees |
6.1 Basic Principles |
6.2 Binary Trees |
6.3 Balanced Trees |
6.4 Threaded Binary Trees |
6.5 Applications of Trees |
7 Graphs and Digraphs |
7.1 Introduction |
7.2 Internal Representation |
7.3 Traversals |
7.4 Spanning Trees |
7.5 Shortest Path Algorithm |
8 Searching |
8.1 Introduction |
8.2 Sequential Searching |
8.3 Searching Ordered Tables |
8.4 Hashing |
9 Sorting Techniques |
9.1 Introduction |
9.2 Bubble Sort |
9.3 Selection Sort |
9.4 Insertion Sort |
9.5 Quicksort |
9.6 Heapsort |
9.7 Mergesort |
Appendix A Acrostic Puzzle |
Appendix B C for Programmers |
B.1 Introduction |
B.2 Data Types |
B.3 Declarations |
B.4 Operator Set |
B.5 Expressions and Statements |
B.6 Control Flow |
B.7 Pointers |
B.8 The C Preprocessor Suggested Readings |
Index |