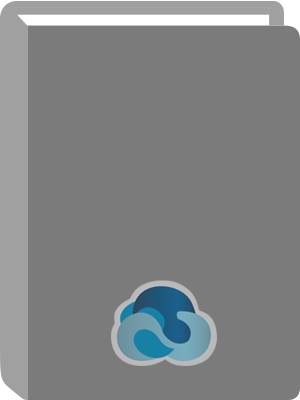
Available:*
Library | Item Barcode | Call Number | Material Type | Item Category 1 | Status |
---|---|---|---|---|---|
Searching... | 30000010328908 | TJ223.P76 M664 2014 | Open Access Book | Book | Searching... |
On Order
Summary
Summary
Take your Arduino skills to the next level!
In this practical guide, electronics guru Simon Monk takes you under the hood of Arduino and reveals professional programming secrets. Featuring coverage of the Arduino Uno, Leonardo, and Due boards, Programming Arduino Next Steps: Going Further with Sketches shows you how to use interrupts, manage memory, program for the Internet, maximize serial communications, perform digital signal processing, and much more. All of the 75+ example sketches featured in the book are available for download.
Learn advanced Arduino programming techniques, including how to:
Use hardware and timer interrupts Boost performance and speed by writing time-efficient sketches Minimize power consumption and memory usage Interface with different types of serial busses, including I2C, 1-Wire, SPI, and TTL Serial Use Arduino with USB, including the keyboard and mouse emulation features of the Leonardo and Due boards Program Arduino for the Internet Perform digital signal processing Accomplish more than one task at a time--without multi-threading Create and release your own code libraryAuthor Notes
Dr. Simon Monk has a degree in Cybernetics and Computer Science and a PhD in Software Engineering. He spent several years as an academic before he returned to industry, co-founding the mobile software company Momote Ltd. Dr. Monk has been an active electronics hobbyist since his early teens and is a full-time writer on hobby electronics and open source hardware. He is the author of numerous electronics books, including Programming the Raspberry Pi: Getting Started with Python; Programming Arduino: Getting Started with Sketches; 30 Arduino Projects for the Evil Genius; Arduino + Android Projects for the Evil Genius , and Practical Electronics for Inventors , Third Edition (co-author).
Table of Contents
Acknowledgments | p. xvii |
Introduction | p. xix |
1 Programming Arduino | p. 1 |
What Is Arduino? | p. 1 |
Installation and the IDE | p. 4 |
Installing the IDE | p. 4 |
Blink | p. 4 |
A Tour of Arduino | p. 7 |
Power Supply | p. 8 |
Power Connections | p. 8 |
Analog Inputs | p. 9 |
Digital Connections | p. 9 |
Arduino Boards | p. 10 |
Uno and Similar | p. 10 |
Big Arduino Boards | p. 11 |
Small Arduino Boards | p. 13 |
LilyPad and LilyPad USB Boards | p. 13 |
Unofficial Arduinos | p. 13 |
Programming Language | p. 15 |
Modifying the Blink Sketch | p. 15 |
Variables | p. 17 |
If | p. 18 |
Loops | p. 19 |
Functions | p. 20 |
Digital Inputs | p. 22 |
Digital Outputs | p. 24 |
The Serial Monitor | p. 24 |
Arrays and Strings | p. 26 |
Analog Inputs | p. 28 |
Analog Outputs | p. 30 |
Using Libraries | p. 32 |
Arduino Data Types | p. 34 |
Arduino Commands | p. 35 |
Summary | p. 36 |
2 Under the Hood | p. 37 |
A Brief History of Arduino | p. 37 |
Anatomy of an Arduino | p. 38 |
AVR Processors | p. 39 |
ATmega328 | p. 39 |
ATmega32u4 | p. 41 |
ATmega2560 | p. 41 |
AT91SAM3X8E | p. 41 |
Arduino and Wiring | p. 42 |
From Sketch to Arduino | p. 46 |
AVR Studio | p. 49 |
Installing a Bootloader | p. 51 |
Burning a Bootloader with AVR Studio and a Programmer | p. 51 |
Burning a Bootloader with the Arduino IDE and a Second Arduino | p. 53 |
Summary | p. 55 |
3 Interrupts and Timers | p. 57 |
Hardware Interrupts | p. 57 |
Interrupt Pins | p. 60 |
Interrupt Modes | p. 61 |
Enabling Internal Pull-Up | p. 61 |
Interrupt Service Routines | p. 62 |
Volatile Variables | p. 63 |
ISR Summary | p. 64 |
Enabling and Disabling Interrupts | p. 64 |
Timer Interrupts | p. 64 |
Summary | p. 68 |
4 Making Arduino Faster | p. 69 |
How Fast Is an Arduino? | p. 69 |
Comparing Arduino Boards | p. 70 |
Speeding Up Arithmetic | p. 71 |
Do You Really Need to Use a Float? | p. 72 |
Lookup vs. Calculate | p. 72 |
Fast I/O | p. 75 |
Basic Code Optimization | p. 75 |
Bytes and Bits | p. 77 |
ATmega328 Ports | p. 77 |
Very Fast Digital Output | p. 79 |
Fast Digital Input | p. 80 |
Speeding Up Analog Inputs | p. 81 |
Summary | p. 83 |
5 Low Power Arduino | p. 85 |
Power Consumption of Arduino Boards | p. 85 |
Current and Batteries | p. 87 |
Reducing the Clock Speed | p. 88 |
Turning Things Off | p. 90 |
Sleeping | p. 92 |
Narcoleptic | p. 92 |
Waking on External Interrupts | p. 94 |
Use Digital Outputs to Control Power | p. 97 |
Summary | p. 99 |
6 Memory | p. 101 |
Arduino Memory | p. 101 |
Minimizing RAM Usage | p. 103 |
Use the Right Data Structures | p. 103 |
Be Careful with Recursion | p. 104 |
Store String Constants in Hash Memory | p. 106 |
Common Misconceptions | p. 106 |
Measure Free Memory | p. 107 |
Minimizing Flash Usage | p. 108 |
Use Constants | p. 108 |
Remove Unwanted Trace | p. 108 |
Bypass the Bootloader | p. 109 |
Static vs. Dynamic Memory Allocation | p. 109 |
Strings | p. 111 |
C char Arrays | p. 111 |
The Arduino String Object Library | p. 114 |
Using EEPROM | p. 115 |
EEPROM Example | p. 116 |
Using the avr/eeprom.h Library | p. 119 |
EEPROM Limitations | p. 121 |
Using Flash | p. 121 |
Using SD Card Storage | p. 123 |
Summary | p. 124 |
7 Using 12C | p. 125 |
12C Hardware | p. 127 |
The 12C Protocol | p. 128 |
The Wire Library | p. 129 |
Initializing 12C | p. 129 |
Master Sending Data | p. 129 |
Master Receiving Data | p. 130 |
12C Examples | p. 131 |
TEA5767 FM Radio | p. 131 |
Arduino-to-Arduino Communication | p. 133 |
LED Backpack Boards | p. 136 |
DS1307 Real-Time Clock | p. 137 |
Summary | p. 139 |
8 Interfacing with 1-Wire Devices | p. 141 |
1-Wire Hardware | p. 141 |
The 1-Wire Protocol | p. 142 |
The OneWire Library | p. 142 |
Initializing 1-Wire | p. 143 |
Scanning the Bus | p. 143 |
Using the DS18B20 | p. 145 |
Summary | p. 147 |
9 Interfacing with SPI Devices | p. 149 |
Bit Manipulation | p. 149 |
Binary and Hex | p. 150 |
Masking Bits | p. 151 |
Shifting Bits | p. 152 |
SPI Hardware | p. 154 |
The SPI Protocol | p. 156 |
The SPI Library | p. 156 |
SPI Example | p. 158 |
Summary | p. 162 |
10 Serial UART Programming | p. 163 |
Serial Hardware | p. 163 |
Serial Protocol | p. 165 |
The Serial Commands | p. 166 |
The SoftwareSerial Library | p. 168 |
Serial Examples | p. 169 |
Computer to Arduino over USB | p. 169 |
Arduino to Arduino | p. 171 |
GPS Module | p. 174 |
Summary | p. 178 |
11 USB Programming | p. 179 |
Keyboard and Mouse Emulation | p. 179 |
Keyboard Emulation | p. 180 |
Keyboard Emulation Example | p. 181 |
Mouse Emulation | p. 182 |
Mouse Emulation Example | p. 182 |
USB Host Programming | p. 183 |
USB Host Shield and Library | p. 183 |
USB Host on the Arduino Due | p. 188 |
Summary | p. 191 |
12 Network Programming | p. 193 |
Networking Hardware | p. 193 |
Ethernet Shield | p. 193 |
Arduino Ethernet/EtherTen | p. 194 |
Arduino and WiFi | p. 194 |
The Ethernet Library | p. 196 |
Making a Connection | p. 196 |
Setting Up a Web Server | p. 199 |
Making Requests | p. 200 |
Ethernet Examples | p. 201 |
Physical Web Server | p. 201 |
Using a JSON Web Service | p. 206 |
The WiFi Library | p. 208 |
Making a Connection | p. 208 |
WiFi Specific Functions | p. 208 |
WiFi Example | p. 209 |
Summary | p. 210 |
13 Digital Signal Processing | p. 211 |
Introducing Digital Signal Processing | p. 211 |
Averaging Readings | p. 213 |
An Introduction to Filtering | p. 215 |
Creating a Simple Low-Pass Filter | p. 215 |
Arduino UnoDSP | p. 217 |
Arduino DueDSP | p. 219 |
Filter Code Generation | p. 221 |
The Fourier Transform | p. 224 |
Spectrum Analyzer Example | p. 226 |
Frequency Measurement Example | p. 228 |
Summary | p. 229 |
14 Managing with One Process | p. 231 |
Making the Transition from Big Programming | p. 231 |
Why You Don't Need Threads | p. 232 |
Setup and Loop | p. 232 |
Sense Then Act | p. 233 |
Pause Without Blocking | p. 234 |
The Timer Library | p. 235 |
Summary | p. 237 |
15 Writing Libraries | p. 239 |
When to Make a Library | p. 239 |
Using Classes and Methods | p. 240 |
Library Example (TEA5767 Radio) | p. 240 |
Define the API | p. 241 |
Write the Header File | p. 243 |
Write the Implementation File | p. 243 |
Write the Keywords File | p. 244 |
Make the Examples Folder | p. 245 |
Testing the Library | p. 246 |
Releasing the Library | p. 246 |
Summary | p. 247 |
A Parts | p. 249 |
Arduino Boards | p. 249 |
Shields | p. 249 |
Components and Modules | p. 250 |
Suppliers | p. 250 |
Index | p. 251 |