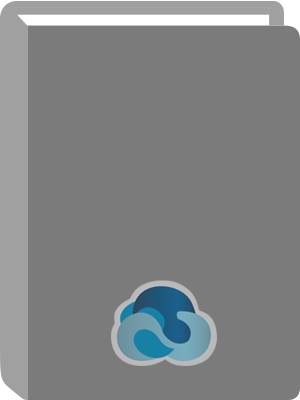
Available:*
Library | Item Barcode | Call Number | Material Type | Item Category 1 | Status |
---|---|---|---|---|---|
Searching... | 30000010128726 | QA76.73.J38 W56 2001 | Open Access Book | Book | Searching... |
Searching... | 30000010122325 | QA76.73.J38 W56 2001 | Open Access Book | Book | Searching... |
On Order
Summary
Summary
This book is written in the clear and concise style that has made Winston's C, C++, and Smalltalk books of the "On To" series so popular. To illuminate the key concepts of Java, the authors add various capabilities to a short yet representative Java program which demonstrates how Java is used for building both stand-alone programs and web applets. Using this book, readers learn Java quickly and effectively, and learn why Java is the language of choice for writing programs for the World Wide Web. On to Java 1.3 offers a clear and concise introduction to the Java programming language. In this third edition, the authors increase coverage of advanced material, explaining how to ensure good programming practice using interfaces, build complex systems using the model-view approach to system design, add sophistication to graphical interfaces with complex tables, define new layout managers for special situations, increase program flexibility using factories, package applications into JAR files, enable program interaction via remote method invocation, and create network services using servlets. This book is designed for programmers familiar with other programming language who need or want to learn the Java language.
Excerpts
Excerpts
HOW THIS BOOK TEACHES YOU THE JAVA PROGRAMMING LANGUAGE 1. The purpose of this book is to help you learn the essentials of Java™programming. In this chapter, you learn about Java's history, Java's special features, and this book's organization. 2. Java was designed for writing programs that run on computers embedded in consumer-electronics appliances, such as microwave ovens and television sets. Accordingly, the design choices made by the developers of Java reflect the expectation that the language would be used to implement small, distributed, and necessarily robust programs. 3. Java has captured the interest and attention of programmers because certain features--conceived in the expectation that programmers would use Java to build programs for consumer electronics--happen to make Java the ideal language for building programs for use on computers connected to the Internet: Java programs run on a wide variety of hardware platforms. Java programs can be loaded dynamically via a network. Java provides features that facilitate robust behavior. In addition, Java has features that make it an excellent language even for applications that have nothing to do with networks: Java is a thoroughly object-oriented language. Java programs can work on multiple tasks simultaneously. Java programs automatically recycle memory. 4. To make Java programs portable, so that they will run on a variety of hardware platforms, the Java compiler translates the programs into byte code. Such translated programs are said to have been compiled into byte code. Programs translated into byte code seem to be written in the instruction set of a typical computer, but byte code is neutral in that it does not employ the instruction set of any particular computer. Instead, byte code is executed by a program that pretends that it is a computer based on the byte-code instruction set. Such a program is called a byte-code interpreter. A byte-code interpreter intended to execute the byte code produced by the Java compiler is called a Java virtual machine. Once a Java virtual machine has been implemented for a particular computer, that computer will run any compiled Java program. Or, said the other way around, any Java application will run on every machine for which a Java virtual machine has been implemented. 5. You might think that byte-code interpretation must mean slow execution, relative to, say, C or C++ programs that are compiled directly into the native instruction set of a particular machine. Fortunately, however, a full-capability Java virtual machine can translate byte code into the native instruction set of the computer through a process called just-in-time compilation. Accordingly, Java programs can run nearly as fast as programs written in older, less portable programming languages. 6. The Java virtual machine helps you to find errors, because the Java virtual machine performs runtime checks that complement the compile-time checks performed by all compilers. For example, when programs are about to access array elements that do not exist, the Java virtual machine displays an informative message and halts, thus catching a common programming error before a hard-to-debug crash occurs. 7. Because Java is object oriented, programs consist of class definitions. In Java, some class definitions establish the characteristics of generic program elements, such as vectors. Other class definitions--the ones that you define yourself--establish the characteristics of application-specific categories, such as railroad cars, stocks, foods, movies, or whatever else happens to come up naturally in your application. Once a class is defined, you can create class instances that describe the particular properties of the individuals that belong to it. When you design a program around classes and class instances, you are said to practice object-oriented programming. In contrast, when you design programs around procedures, you are said to practice procedure-oriented programming. 8. In this book, you learn more about what object oriented means and why many programmers prefer object-oriented languages. For now, it suffices for you to know that Java is a thoroughly object-oriented programming language; in contrast, most programming languages, such as C, are procedure-oriented, and other programming languages, such as C++, are half-procedure-oriented, half-object-oriented hybrids. 9. When you run a Java program, it fetches each class definition only when needed. Thus, Java is said to load classes dynamically or on demand. Certain Java programs are intended to be used in cooperation with a web browser such as Netscape Navigator™, which contains a Java virtual machine capable of loading Java classes dynamically via a local-area network or via the Internet. 10. Java applications loaded by web browsers are based on applet classes. Applet is a word that means little application; however, some applications loaded by web browsers are large. 11. Distributing software via web browsers is wonderfully effective: No programs have to be downloaded and manually installed, and, better still, no disks need to be packaged for sale and no update or bug-fix disks need to be mailed. 12. Another distinguishing feature of Java is that Java allows you to create multiple threads. Each thread is like a separate program in that a thread seems to run at the same time as other threads; in contrast, however, the threads in one program share the same memory. By exploiting the thread feature, you can write programs in which one thread is working through complex statistical formulas, another thread is fetching data from a file, still other thread is transmitting data over a network, and yet another thread is updating a play. All-these threads share your computer's time. Because no thread ever has to wait for mother thread to finish a task, you can write programs that exhibit an extraordinarily responsive look and feel. 13. Java increases your productivity by providing automatic memory recycling. When you use a language such as C++, you have to remember to free the memory allocated to program elements, such as class instances, once you are finished with them. Failing to free memory produces a memory leak that may exhaust all the memory available to your program, leading either to erratic behavior or to a total program crash. Java frees memory automatically, by performing automatic garbage collection, so you never need worry about memory leaks or waste time looking for one. Thus, you are more productive, and less likely to be driven crazy via tedious, mind-numbing debugging. Most programming languages do not offer garbage collection, even though languages such as Lisp and Smalltalk established the great value of garbage collection decades ago. 14. In addition to automatic garbage collection, the Java virtual machine provides a variety of other features that facilitate secure behavior. For example, no Java program, run via a web browser, can open, read, or write files on your computer; it is therefore difficult for someone to corrupt or hobble your software, either deliberately or inadvertently. 15. For many programmers, Java is easy to learn because Java's syntax is largely based on that of the popular C and C++ programming languages. Although Java programs resemble C and C++ programs when viewed at a distance, the Java programming language excludes many of the characteristics of C and C++ believed by Java's designers to harm program readability and robustness. For example, Java programmers do not think in terms of pointers and they do not overload operators. 16. To get you up and running in Java quickly, the chapters in this book generally supply you with the most useful approach to each programming need, be it to display characters on your screen, to define a new method, or to read information from a file. To help you acquire the knowledge you need quickly, this book is divided into chapters that generally focus on one question, which is plainly announced in the title of the chapter. Accordingly, you see titles such as the following: How to Compile and Run a Simple Program How to Define Constructor Instance Methods How to Benefit from Data Abstraction How to Define Abstract Classes and Abstract Methods How to Design Classes and Class Hierarchies How to Modularize Programs Using Compilation Units and Packages How to Enforce Requirements and to Document Programs Using Interfaces How to Use the Model-View Approach to Interface Design How to Access Applets from Web Browsers How to Use Threads to Implement Dynamic Applets How to Use Resource Locators to Access Files How to Collect Information Using Servlets 18. So that you are encouraged to develop a personal library of solutions to standard programming problems, this book introduces many useful, productivity-increasing, general-purpose, template-like patterns that you can fill in to achieve particular-purpose goals. These template-like patterns are often called programming idioms. You learn about programming idioms because learning to program involves more than learning to use rules of program composition, just as learning to speak a human language involves more than learning to use vocabulary words. 19. So that you can deepen your understanding of the art of good programming practice, this book emphasizes the value of powerful ideas, such as procedure abstraction and data abstraction; introduces key mechanisms, such as the interface mechanism for imposing requirements and encouraging documentation; and explains important principles, such as the explicit-representation principle, no-duplication principle, lookup principle, and need-to-know principle. 20. In this book, single-idea segments, analogous to slides, are arranged in chapters that are analogous to slide shows. There are several segment varieties: mainline segments explain essential ideas; sidetrip segments introduce interesting, but skippable, ideas; practice segments provide opportunities to experiment with new ideas; and highlights segments summarize important points. 21. The book develops a simple, yet realistic Java program, which you see in many versions as your understanding of the language increases. In its ultimate version, the program reads from a file that contains information about movies, computes an overall rating for a selected movie, displays the rating on a meter, and shows an advertising poster, if one is available. The program runs either as a standalone application or as an applet meant to be used via a network viewer. The applet version presents the following appearance: 22. Highlights Its features make Java ideally suited for writing network-oriented programs. Java is an object-oriented programming language. When you use an object-oriented programming language, your programs consist of class definitions. Java class definitions and the programs associated with classes are compiled into byte code, which facilitates program portability. Java class definitions and the programs associated with them can be loaded dynamically via a network. Java's compiler detects errors at compile timer; the Java virtual machine detects errors at run time. Java programs can be multithreaded, thereby enabling them to perform many tasks simultaneously. Java programs collect garbage automatically, relieving you of tedious programming and frustrating debugging, thereby increasing your productivity. Java has synatical similarities with the C and C++ languages. This book introduces and emphasizes powerful ideas, key mechanisms, and important principles. Excerpted from On to Java by Patrick Henry Winston, Sundar Narasimhan All rights reserved by the original copyright owners. Excerpts are provided for display purposes only and may not be reproduced, reprinted or distributed without the written permission of the publisher.Table of Contents
How this Book Teaches you the Java Programming Language |
How To Benefit from Procedure Abstraction |
How To Declare Class Variables |
How To Create Class Instances |
How To Define Instance Methods |
How To Define Constructors |
How To Define Getter and Setter Methods |
How To Benefit from Data Abstraction |
How To Define Classes that Inherit Instance Variables and Methods |
How To Enforce Abstraction Using Protected and Private Variables and Methods |
How To Write Constructors that Call other Constructors |
How To Write Methods that Call other Methods |
How To Design Classes and Class Heirarchies |
How To Enforce Requirements Using Abstract Classes and Abstract Methods |
How To Enforce Requirements and to Document Programs Using Interfaces |
How To Perform Tests Using Predicates |
How To Write Conditional Statements |
How To Combine Boolean Expressions |
How To Write Iteration Statements |
How To Write Recursive Methods |
How To Write Multiway Conditional Statements |
How To Work with File Input Streams |
How To Create and Access Arrays |
How To Move Arrays into and out of Methods |
How To Store Data in Expandable Vectors |
How To Work with Characters and Strings |
How To Catch Exceptions |
How To Work with Output File Streams |
How To Write and Read Values Using the Serializable Interface |
How To Modularize Programs using Compilation Units and Packages |
How To Combine Private Variables and Methods with Packages |
How To Create Windows and to Activate Listeners |
How To Define Inner Classes and to Structure Applications |
How To Draw Lines in Windows |
How To Write Text in Windows |
How To Use the Model - View Approach to GUI Design |
How To Define Standalone Observers and Listeners. |
How To Define Applets |
How To Access Applets from Web Browsers |
How To Use Resource Locators |
How To Use Choice Lists To Select Instances |
How To Bring Images into Applets |
How To Use Threads To Implement Dynamic Applets |
How To Create Forms and to Fire Your Own Events |
How To Display Menus and File Dialog Windows |
How To Develop Your Own Layout Manager |
How To Implement Dynamic Tables |
How To Activate Remote Computations |
How To Collect Information Using Servlets |
How To Construct Jar Files for Program Distribution |
Appendix A Operator Precedence |
Appendix B The Meter Canvas |
Appendix C Applet Parameters |
Appendix D The Swing Classes |
Appendix E Layout Managers |
Appendix F The Graphics2d Package |
Colophon |
Software |
Books. |