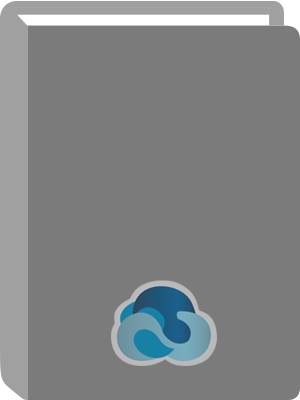
Available:*
Library | Item Barcode | Call Number | Material Type | Item Category 1 | Status |
---|---|---|---|---|---|
Searching... | 30000004533711 | QA76.6 K48 2000 | Open Access Book | Book | Searching... |
On Order
Summary
Summary
This book presents concepts of programming methodology and sound software development alongside the fundamentals of the Visual Basic 6.0 language. The goal is to provide a foundation of solid programming techniques and to promote an understanding of the common control structures available in most high-level languages. The book discusses the language with gradually increasing complexity, presenting the essential features of Visual Basic before introducing advanced language features. This is an appropriate book for introductory courses in computer programming as well as a reference for advanced programmers. Features: *Provides a solid foundation in computer programming fundamentals using the Visual Basic language *Contains well thought-out pedagogy, including: -Code Callouts to explain important points and key concepts in program source code -GUI Design Tips to enhance understanding of proper GUI design -Real-world examples from the business, math, science, engineering, and operations research communities to demonstrate the relevance of the material -Case Studies to provide insight on how the concepts apply to real-world situations -Chapter Summaries to review key terms, words, and c
Table of Contents
Preface | p. vii |
1 Computer Basics | p. 1 |
Chapter Objectives | p. 1 |
A Brief History of Computers | p. 1 |
Types of Computers | p. 7 |
Components of a Typical Microcomputer System | p. 8 |
The Binary Number System | p. 9 |
The Evolution of Computer Programming Languages | p. 10 |
From BASIC to Visual Basic | p. 11 |
Summary | p. 13 |
Key Terms | p. 13 |
Key Concepts | p. 14 |
Review Questions | p. 15 |
Problems | p. 15 |
Next Step Visual Basic: Computer Arithmetic and Number Systems | p. 16 |
Chapter Supplement Objectives | p. 16 |
A Look Back at the Decimal Number System | p. 16 |
The Binary Number System | p. 17 |
Binary Arithmetic | p. 19 |
Octal and Hexadecimal Number Systems | p. 21 |
Summary | p. 23 |
Key Terms | p. 23 |
Key Concepts | p. 24 |
Review Questions | p. 24 |
Supplement Problems | p. 24 |
2 The Visual Basic Development Environment | p. 27 |
Chapter Objectives | p. 27 |
The Visual Basic Philosophy | p. 28 |
Using Visual Basic | p. 28 |
The Visual Basic Opening Screen | p. 29 |
Visual Basic Screen Layout | p. 29 |
Elementary Visual Basic Controls | p. 33 |
Label | p. 34 |
Text Box | p. 35 |
Picture Box | p. 37 |
Command Button | p. 37 |
Placing Moving and Sizing Controls | p. 37 |
Accessing Additional Toolbox Controls | p. 38 |
Control Naming Conventions | p. 38 |
Changing the Integrated Development Environment | p. 38 |
Your First Program | p. 39 |
Designing the User Interface | p. 41 |
Writing the Source Code | p. 41 |
Visual Basic File Types | p. 42 |
Visual Basic Help and Online Documentation | p. 45 |
Summary | p. 47 |
Key Terms | p. 47 |
Keywords | p. 48 |
Key Concepts | p. 48 |
Review Questions | p. 49 |
Problems | p. 49 |
Next Step Visual Basic: Customizing the Visual Basic Integrated Development Environment | p. 51 |
Chapter Supplement Objectives | p. 51 |
Editor Tab | p. 51 |
Editor Format Tab | p. 52 |
General Tab | p. 53 |
Docking Tab | p. 54 |
Environment Tab | p. 55 |
Advanced Tab | p. 56 |
Summary | p. 57 |
Key Terms | p. 57 |
Key Concepts | p. 57 |
Review Questions | p. 58 |
Supplement Problems | p. 58 |
3 Planning Your Program | p. 59 |
Chapter Objectives | p. 59 |
Planning: The First Step | p. 60 |
Flowcharts | p. 60 |
Pseudocode | p. 61 |
The Program Development Cycle | p. 62 |
Case Study: The Knapsack Problem | p. 63 |
Summary | p. 65 |
Key Terms | p. 65 |
Key Concepts | p. 65 |
Review Questions | p. 65 |
Problems | p. 65 |
4 Elements of Programming | p. 69 |
Chapter Objectives | p. 69 |
Variables | p. 69 |
Naming Declaring and Using Variables | p. 70 |
Code Views | p. 72 |
Declaring Variables | p. 72 |
Variant Data Type | p. 73 |
Using = in Code | p. 74 |
Initializing Variables | p. 74 |
Constants | p. 75 |
Defining Constants | p. 75 |
Mathematical Calculations | p. 75 |
Order of Operations | p. 77 |
Mathematical Functions | p. 78 |
Mixed-Mode Arithmetic | p. 78 |
Strings | p. 80 |
String Concatenation | p. 80 |
Special Characters in Strings | p. 80 |
String Functions | p. 81 |
Fixed-Length Strings | p. 81 |
Scope and Lifetime | p. 82 |
Program Readability | p. 84 |
Comment Keywords | p. 84 |
Line Continuation Character | p. 85 |
Interactive Input and Output | p. 85 |
Text Boxes | p. 85 |
Picture Boxes | p. 85 |
Input Boxes and Message Boxes | p. 86 |
Your Second Program | p. 87 |
CASE STUDY: The Global Variable Trap | p. 90 |
Summary | p. 90 |
Key Terms | p. 90 |
Keywords | p. 92 |
Key Concepts | p. 93 |
Review Questions | p. 94 |
Problems | p. 95 |
Programming Projects | p. 96 |
5 Flow Control | p. 97 |
Chapter Objectives | p. 97 |
Comparison Operators | p. 98 |
Relational Operators | p. 98 |
Logical Operators | p. 99 |
Relational and Logical Operator Precedence | p. 99 |
Logical Expressions | p. 99 |
DeMorgan's Laws | p. 100 |
Expanding and Reducing Logical Expressions | p. 100 |
Decision Structures | p. 102 |
If-Blocks | p. 102 |
Comparison of Code Structures | p. 104 |
Nested If-Blocks | p. 104 |
Select-Case Blocks | p. 105 |
Repetition Structures | p. 107 |
For-Next Loops | p. 107 |
Do Loops | p. 110 |
Comparing Do While-Loop and Do-Loop Until | p. 111 |
Comparative Example of Do Loops | p. 111 |
Other Do Loops | p. 112 |
Ending a Program | p. 112 |
Programming Style | p. 113 |
CASE STUDY: GoodLook Cosmetics Company | p. 113 |
Summary | p. 114 |
Key Terms | p. 114 |
Keywords | p. 115 |
Key Concepts | p. 116 |
Review Questions | p. 118 |
Problems | p. 118 |
Programming Projects | p. 120 |
Next Step Visual Basic: An Introduction to Sequential File Input and Output | p. 121 |
Supplement Objectives | p. 121 |
Sequential Files | p. 121 |
Summary | p. 123 |
Key Terms | p. 123 |
Keywords | p. 124 |
Key Concepts | p. 124 |
Review Questions | p. 124 |
Supplement Problems | p. 125 |
Programming Projects | p. 125 |
6 Structured Programming | p. 127 |
Chapter Objectives | p. 127 |
Modular Programming | p. 128 |
Subprograms: Procedures and Functions | p. 128 |
An Example of a Subprogram | p. 128 |
Arguments and Parameters | p. 129 |
Defining and Using Subprograms | p. 130 |
Procedures | p. 130 |
Functions | p. 131 |
Creating a Subprogram in VB | p. 132 |
Parameter Passing | p. 132 |
Events and Event Handlers | p. 135 |
Even Handlers for Form Events | p. 135 |
Event Handlers for Focus Events | p. 136 |
Event Handlers for Mouse Events | p. 136 |
Summary | p. 138 |
Key Terms | p. 138 |
Keywords | p. 139 |
Key Concepts | p. 139 |
Review Questions | p. 140 |
Problems | p. 141 |
Programming Projects | p. 142 |
Next Step Visual Basic: Recursion | p. 144 |
Supplement Objectives | p. 144 |
Mathematical Recursion | p. 144 |
Recursive Subprograms | p. 145 |
CASE STUDY: The Towers of Hanoi | p. 146 |
Summary | p. 149 |
Key Terms | p. 149 |
Key Concepts | p. 149 |
Review Questions | p. 149 |
Supplement Problems | p. 149 |
Programming Projects | p. 150 |
7 Error Trapping and Debugging | p. 151 |
Chapter Objectives | p. 151 |
Built-In Error Trapping | p. 152 |
The GoTo Statement | p. 154 |
Types of Programming Errors | p. 155 |
Syntax Errors and Logic Errors | p. 155 |
Standard Debugging Techniques | p. 156 |
Data Dump | p. 156 |
Hand-Execution of Code | p. 156 |
The Visual Basic Debugger | p. 157 |
Debugger Operation | p. 159 |
VB Debugger Example | p. 159 |
Case Study: A Failed Space Mission | p. 166 |
Case Study: The Y2K Problem | p. 166 |
Summary | p. 167 |
Key Terms | p. 167 |
Keywords | p. 168 |
Key Concepts | p. 168 |
Review Questions | p. 169 |
Problems | p. 170 |
8 Advanced Data Structures | p. 173 |
Chapter Objectives | p. 173 |
Static vs. Dynamic Data Structures | p. 173 |
Arrays | p. 174 |
The Option Base Statement | p. 176 |
Accessing an Array Element | p. 177 |
Array Structures and Notation | p. 178 |
Dynamic Arrays | p. 179 |
Arrays as Arguments and Parameters | p. 180 |
CASE STUDY: Using Arrays | p. 182 |
Records and User-Defined Data Types | p. 185 |
Records | p. 186 |
User-Defined Data Types | p. 186 |
Using Records | p. 187 |
Stacks, Queues, Deques, and Lists | p. 189 |
Stacks | p. 189 |
Queues | p. 192 |
Deques | p. 194 |
Pointers and Linked Lists | p. 198 |
Linked List Example | p. 199 |
Summary | p. 202 |
Key Terms | p. 202 |
Keywords | p. 203 |
Key Concepts | p. 203 |
Review Questions | p. 205 |
Problems | p. 205 |
Programming Projects | p. 205 |
Next Step Visual Basic: Object-Oriented Programming | p. 207 |
Chapter Supplement Objectives | p. 207 |
Overview of Object-Oriented Programming | p. 207 |
An Example Object-Oriented Program in VB | p. 209 |
Summary | p. 214 |
Key Terms | p. 214 |
Keywords | p. 214 |
Key Concepts | p. 214 |
Review Questions | p. 216 |
Supplement Problems | p. 216 |
Programming Projects | p. 216 |
9 File Input and Output | p. 217 |
Chapter Objectives | p. 217 |
Sequential Files | p. 218 |
CASE STUDY: Character Pattern Matching | p. 222 |
Random-Access Files | p. 223 |
Summary | p. 228 |
Key Terms | p. 228 |
Keywords | p. 229 |
Key Concepts | p. 229 |
Review Questions | p. 230 |
Problems | p. 230 |
Programming Projects | p. 231 |
Next Step Visual Basic: Sorting and Searching | p. 232 |
Chapter Supplement Objectives | p. 232 |
Sorting | p. 232 |
Searching | p. 237 |
Summary | p. 238 |
Key Terms | p. 238 |
Key Concepts | p. 239 |
Review Questions | p. 239 |
Supplement Problems | p. 239 |
Programming Projects | p. 240 |
10 Advanced Visual Basic | p. 241 |
Chapter Objectives | p. 241 |
Additional Visual Basic Controls | p. 242 |
Check Box | p. 242 |
Option Button | p. 243 |
Frame | p. 244 |
List Box | p. 245 |
Combo Box | p. 247 |
Horizontal and Vertical Scroll Bars | p. 249 |
Drive Directory and File List Boxes | p. 250 |
Timer | p. 251 |
Shape and Line Controls | p. 253 |
Image | p. 254 |
Data | p. 254 |
OLE | p. 255 |
Common Dialog Control | p. 255 |
Menu Control | p. 256 |
Control Arrays | p. 259 |
ActiveX Controls | p. 262 |
An Active Control Example | p. 262 |
Testing the Actual Control | p. 264 |
Collections | p. 265 |
Multiple Forms Programming and Multiple Document Interface (MDI) Forms | p. 269 |
MDI versus SDI | p. 270 |
MDI Application Example | p. 271 |
Random Numbers | p. 272 |
Graphics | p. 275 |
Object Linking and Embedding (OLE) | p. 278 |
Dynamic Data Exchange (DDE) | p. 279 |
Internet Programming | p. 282 |
Visual Basic Compiler Directives | p. 284 |
CASE STUDY: A Computerized Survey | p. 286 |
Summary | p. 290 |
Key Terms | p. 290 |
Keywords | p. 291 |
Key Concepts | p. 292 |
Review Questions | p. 294 |
Problems | p. 295 |
Programming Projects | p. 295 |
Next Step Visual Basic: Package and Deployment Wizard | p. 296 |
Chapter Supplement Objectives | p. 296 |
Using the Package Deployment Wizard | p. 296 |
Using the Package and Deployment Wizard as a VB Add-In | p. 297 |
Summary | p. 300 |
Key Terms | p. 300 |
Key Concepts | p. 300 |
Review Questions | p. 301 |
Supplement Problems | p. 301 |
Programming Projects | p. 301 |
11 Visual Basic for Applications | p. 303 |
Chapter Objectives | p. 303 |
Using Macros | p. 304 |
An Example Macro in Microsoft Word | p. 305 |
An Example Macro in Microsoft Excel | p. 308 |
Using Macro Shortcuts | p. 312 |
Using Visual Basic Control Objects in Microsoft Office Applications | p. 315 |
Differences between Visual Basic and VBA | p. 319 |
Case Study: A Loan Amortization Table | p. 320 |
Summary | p. 325 |
Key Terms | p. 325 |
Key Concepts | p. 325 |
Review Questions | p. 325 |
Problems | p. 326 |
Programming Projects | p. 326 |
12 Databases | p. 327 |
Chapter Objectives | p. 327 |
Database Fundamentals | p. 327 |
Relational Database Design | p. 328 |
Database Engine | p. 329 |
Creating Database Files | p. 330 |
Visual Data Manager | p. 330 |
Using Data Controls and Data-Aware Controls | p. 334 |
Structured Query Language (SQL) | p. 340 |
SQL Overview | p. 340 |
Using the Select Command in SQL | p. 340 |
Creating a Database through Visual Basic Code | p. 343 |
Case Study: Designing a Relational Database | p. 347 |
Summary | p. 349 |
Key Terms | p. 349 |
Keywords | p. 350 |
SQL Keywords | p. 351 |
Key Concepts | p. 351 |
Review Questions | p. 352 |
Problems | p. 352 |
Programming Projects | p. 353 |
Appendix A ASCII (ANSI) Character Values | p. 355 |
Appendix B Visual Basic Programming Standards | p. 357 |
Appendix C Visual Basic Trappable Errors | p. 361 |
Appendix D Visual Basic Keyword Summary | p. 364 |
Index | p. 369 |