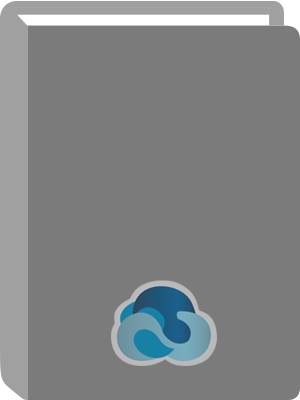
Available:*
Library | Item Barcode | Call Number | Material Type | Item Category 1 | Status |
---|---|---|---|---|---|
Searching... | 30000010263544 | QA76.73 .C153 D45 2012 | Open Access Book | Book | Searching... |
On Order
Summary
Summary
For Introduction to Programming (CS1) and other more intermediate courses covering programming in C++. Also appropriate as a supplement for upper-level courses where the instructor uses a book as a reference for the C++ language.
This best-selling comprehensive text is aimed at readers with little or no programming experience. It teaches programming by presenting the concepts in the context of full working programs and takes an early-objects approach. The authors emphasize achieving program clarity through structured and object-oriented programming, software reuse and component-oriented software construction. The Eighth Edition encourages students to connect computers to the community, using the Internet to solve problems and make a difference in our world. All content has been carefully fine-tuned in response to a team of distinguished academic and industry reviewers.
Author Notes
Paul J. Deitel , CEO and Chief Technical Officer of Deitel & Associates, Inc., is a graduate of MIT's Sloan School of Management, where he studied Information Technology. He holds the Java Certified Programmer and Java Certified Developer certifications, and has been designated by Sun Microsystems as a Java Champion. Through Deitel & Associates, Inc., he has delivered Java, C, C++, C# and Visual Basic courses to industry clients, including IBM, Sun Microsystems, Dell, Lucent Technologies, Fidelity, NASA at the Kennedy Space Center, the National Severe Storm Laboratory, White Sands Missile Range, Rogue Wave Software, Boeing, Stratus, Cambridge Technology Partners, Open Environment Corporation, One Wave, Hyperion Software, Adra Systems, Entergy, CableData Systems, Nortel Networks, Puma, iRobot, Invensys and many more. He has also lectured on Java and C++ for the Boston Chapter of the Association for Computing Machinery. He and his father, Dr. Harvey M. Deitel, are the world's best-selling programming language textbook authors.
Dr. Harvey M. Deitel , Chairman and Chief Strategy Officer of Deitel & Associates, Inc., has 45 years of academic and industry experience in the computer field. Dr. Deitel earned B.S. and M.S. degrees from the MIT and a Ph.D. from Boston University. He has 20 years of college teaching experience, including earning tenure and serving as the Chairman of the Computer Science Department at Boston College before founding Deitel & Associates, Inc., with his son, Paul J. Deitel. He and Paul are the co-authors of several dozen books and multimedia packages and they are writing many more. With translations published in Japanese, German, Russian, Spanish, Traditional Chinese, Simplified Chinese, Korean, French, Polish, Italian, Portuguese, Greek, Urdu and Turkish, the Deitels' texts have earned international recognition. Dr. Deitel has delivered hundreds of professional seminars to major corporations, academic institutions, government organizations and the military.
Table of Contents
Preface | p. xxi |
1 Introduction to Computers and C++ | p. 1 |
1.1 Introduction | p. 2 |
1.2 Computers: Hardware and Software | p. 5 |
1.3 Data Hierarchy | p. 6 |
1.4 Computer Organization | p. 7 |
1.5 Machine Languages, Assembly Languages and High-Level Languages | p. 9 |
1.6 Introduction to Object Technology | p. 10 |
1.7 Operating Systems | p. 13 |
1.8 Programming Languages | p. 15 |
1.9 C++ and a Typical C++ Development Environment | p. 17 |
1.10 Test-Driving a C++ Application | p. 21 |
1.11 Web 2.0: Going Social | p. 27 |
1.12 Software Technologies | p. 29 |
1.13 Future of C++: TR1, the New C++ Standard and the Open Source Boost Libraries | p. 31 |
1.14 Keeping Up-to-Date with Information Technologies | p. 32 |
1.15 Wrap-Up | p. 32 |
2 Introduction to C++ Programming | p. 37 |
2.1 Introduction | p. 38 |
2.2 First Program in C++: Printing a Line of Text | p. 38 |
2.3 Modifying Our First C++ Program | p. 42 |
2.4 Another C++ Program: Adding Integers | p. 43 |
2.5 Memory Concepts | p. 47 |
2.6 Arithmetic | p. 48 |
2.7 Decision Making: Equality and Relational Operators | p. 51 |
2.8 Wrap-Up | p. 55 |
3 Introduction to Classes, Objects and Strings | p. 64 |
3.1 Introduction | p. 65 |
3.2 Defining a Class with a Member Function | p. 65 |
3.3 Defining a Member Function with a Parameter | p. 68 |
3.4 Data Members, set Functions and get Functions | p. 71 |
3.5 Initializing Objects with Constructors | p. 77 |
3.6 Placing a Class in a Separate File for Reusability | p. 81 |
3.7 Separating Interface from Implementation | p. 84 |
3.8 Validating Data with set Functions | p. 90 |
3.9 Wrap-Up | p. 95 |
4 Control Statements: Part1 | p. 101 |
4.1 Introduction | p. 102 |
4.2 Algorithms | p. 102 |
4.3 Pseudocode | p. 103 |
4.4 Control Structures | p. 104 |
4.5 if Selection Statement | p. 107 |
4.6 if...else Double-Selection Statement | p. 108 |
4.7 while Repetition Statement | p. 113 |
4.8 Formulating Algorithms: Counter-Controlled Repetition | p. 114 |
4.9 Formulating Algorithms: Sentinel-Controlled Repetition | p. 120 |
4.10 Formulating Algorithms: Nested Control Statements | p. 130 |
4.11 Assignment Operators | p. 134 |
4.12 Increment and Decrement Operators | p. 135 |
4.13 Wrap-Up | p. 138 |
5 Control Statements: Part2 | p. 152 |
5.1 Introduction | p. 153 |
5.2 Essentials of Counter-Controlled Repetition | p. 153 |
5.3 for Repetition Statement | p. 155 |
5.4 Examples Using the for Statement | p. 158 |
5.5 do...while Repetition Statement | p. 162 |
5.6 switch Multiple-Selection Statement | p. 164 |
5.7 break and continue Statements | p. 173 |
5.8 Logical Operators | p. 174 |
5.9 Confusing the Equality (==) and Assignment (=) Operators | p. 179 |
5.10 Structured Programming Summary | p. 180 |
5.11 Wrap-Up | p. 185 |
6 Functions and an Introduction to Recursion | p. 194 |
6.1 Introduction | p. 195 |
6.2 Program Components in C++ | p. 196 |
6.3 Math Library Functions | p. 197 |
6.4 Function Definitions with Multiple Parameters | p. 198 |
6.5 Function Prototypes and Argument Coercion | p. 203 |
6.6 C++ Standard Library Headers | p. 205 |
6.7 Case Study: Random Number Generation | p. 207 |
6.8 Case Study: Game of Chance; Introducing enum | p. 212 |
6.9 Storage Classes | p. 215 |
6.10 Scope Rules | p. 218 |
6.11 Function Call Stack and Activation Records | p. 221 |
6.12 Functions with Empty Parameter Lists | p. 225 |
6.13 Inline Functions | p. 225 |
6.14 References and Reference Parameters | p. 227 |
6.15 Default Arguments | p. 231 |
6.16 Unary Scope Resolution Operator | p. 232 |
6.17 Function Overloading | p. 234 |
6.18 Function Templates | p. 236 |
6.19 Recursion | p. 239 |
6.20 Example Using Recursion: Fibonacci Series | p. 242 |
6.21 Recursion vs. Iteration | p. 245 |
6.22 Wrap-Up | p. 248 |
7 Arrays and Vectors | p. 267 |
7.1 Introduction | p. 268 |
7.2 Arrays | p. 269 |
7.3 Declaring Arrays | p. 270 |
7.4 Examples Using Arrays | p. 271 |
7.4.1 Declaring an Array and Using a Loop to Initialize the Array's Elements | p. 271 |
7.4.2 Initializing an Array in a Declaration with an Initializer List | p. 272 |
7.4.3 Specifying an Array's Size with a Constant Variable and Setting Array Elements with Calculations | p. 273 |
7.4.4 Summing the Elements of an Array | p. 275 |
7.4.5 Using Bar Charts to Display Array Data Graphically | p. 276 |
7.4.6 Using the Elements of an Array as Counters | p. 277 |
7.4.7 Using Arrays to Summarize Survey Results | p. 278 |
7.4.8 Static Local Arrays and Automatic Local Arrays | p. 281 |
7.5 Passing Arrays to Functions | p. 283 |
7.6 Case Study: Class GradeBook Using an Array to Store Grades | p. 287 |
7.7 Searching Arrays with Linear Search | p. 293 |
7.8 Sorting Arrays with Insertion Sort | p. 294 |
7.9 Multidimensional Arrays | p. 297 |
7.10 Case Study: Class GradeBook Using a Two-Dimensional Array | p. 300 |
7.11 Introduction to C++ Standard Library Class Template vector | p. 307 |
7.12 Wrap-Up | p. 313 |
8 Pointers | p. 330 |
8.1 Introduction | p. 331 |
8.2 Pointer Variable Declarations and Initialization | p. 331 |
8.3 Pointer Operators | p. 332 |
8.4 Pass-by-Reference with Pointers | p. 335 |
8.5 Using const with Pointers | p. 339 |
8.6 Selection Sort Using Pass-by-Reference | p. 343 |
8.7 sizeof Operator | p. 347 |
8.8 Pointer Expressions and Pointer Arithmetic | p. 349 |
8.9 Relationship Between Pointers and Arrays | p. 352 |
8.10 Pointer-Based String Processing | p. 354 |
8.11 Arrays of Pointers | p. 357 |
8.12 Function Pointers | p. 358 |
8.13 Wrap-Up | p. 361 |
9 Classes: A Deeper Look, Part1 | p. 379 |
9.1 Introduction | p. 380 |
9.2 Time Class Case Study | p. 381 |
9.3 Class Scope and Accessing Class Members | p. 388 |
9.4 Separating Interface from Implementation | p. 389 |
9.5 Access Functions and Utility Functions | p. 390 |
9.6 Time Class Case Study: Constructors with Default Arguments | p. 393 |
9.7 Destructors | p. 398 |
9.8 When Constructors and Destructors Are Called | p. 399 |
9.9 Time Class Case Study: A Subtle Trap-Returning a Reference to a private Data Member | p. 402 |
9.10 Default Memberwise Assignment | p. 405 |
9.11 Wrap-Up | p. 407 |
10 Classes: A Deeper Look, Part2 | p. 414 |
10.1 Introduction | p. 415 |
10.2 const (Constant) Objects and const Member Functions | p. 415 |
10.3 Composition: Objects as Members of Classes | p. 423 |
10.4 friend Functions and friend Classes | p. 429 |
10.5 Using the this Pointer | p. 431 |
10.6 static Class Members | p. 436 |
10.7 Proxy Classes | p. 441 |
10.8 Wrap-Up | p. 445 |
11 Operator Overloading; Class string | p. 451 |
11.1 Introduction | p. 452 |
11.2 Using the Overloaded Operators of Standard Library Class string | p. 453 |
11.3 Fundamentals of Operator Overloading | p. 456 |
11.4 Overloading Binary Operators | p. 457 |
11.5 Overloading the Binary Stream Insertion and Stream Extraction Operators | p. 458 |
11.6 Overloading Unary Operators | p. 462 |
11.7 Overloading the Unary Prefix and Postfix ++ and -- Operators | p. 463 |
11.8 Case Study: A Date Class | p. 464 |
11.9 Dynamic Memory Management | p. 469 |
11.10 Case Study: Array Class | p. 471 |
11.10.1 Using the Array Class | p. 472 |
11.10.2 Array Class Definition | p. 475 |
11.11 Operators as Member Functions vs. Non-Member Functions | p. 483 |
11.12 Converting between Types | p. 483 |
11.13 explicit Constructors | p. 485 |
11.14 Building a String Class | p. 487 |
11.15 Wrap-Up | p. 488 |
12 Object-Oriented Programming: Inheritance | p. 499 |
12.1 Introduction | p. 500 |
12.2 Base Classes and Derived Classes | p. 500 |
12.3 protected Members | p. 503 |
12.4 Relationship between Base Classes and Derived Classes | p. 503 |
12.4.1 Creating and Using a CommissionEmployee Class | p. 504 |
12.4.2 Creating a BasePlusCommissionEmployee Class Without Using Inheritance | p. 508 |
12.4.3 Creating a CommissionEmployee-BasePlusCommissionEmployee Inheritance Hierarchy | p. 514 |
12.4.4 CommissionEmployee-BasePlusCommissionEmployee Inheritance Hierarchy Using protected Data | p. 519 |
12.4.5 CommissionEmployee BasePlusCommissionEmployee Inheritance Hierarchy Using private Data | p. 522 |
12.5 Constructors and Destructors in Derived Classes | p. 527 |
12.6 public, protected and private Inheritance | p. 527 |
12.7 Software Engineering with Inheritance | p. 528 |
12.8 Wrap-Up | p. 529 |
13 Object-Oriented Programming: Polymorphism | p. 534 |
13.1 Introduction | p. 535 |
13.2 Introduction to Polymorphism: Polymorphic Video Game | p. 536 |
13.3 Relationships Among Objects in an Inheritance Hierarchy | p. 536 |
13.3.1 Invoking Base-Class Functions from Derived-Class Objects | p. 537 |
13.3.2 Aiming Derived-Class Pointers at Base-Class Objects | p. 540 |
13.3.3 Derived-Class Member-Function Calls via Base-Class Pointers | p. 541 |
13.3.4 Virtual Functions | p. 543 |
13.4 Type Fields and switch Statements | p. 549 |
13.5 Abstract Classes and Pure virtual Functions | p. 549 |
13.6 Case Study: Payroll System Using Polymorphism | p. 551 |
13.6.1 Creating Abstract Base Class Employee | p. 552 |
13.6.2 Creating Concrete Derived Class Salaried Employee | p. 556 |
13.6.3 Creating Concrete Derived Class Commission Employee | p. 558 |
13.6.4 Creating Indirect Concrete Derived Class Base Plus Commission Employee | p. 560 |
13.6.5 Demonstrating Polymorphic Processing | p. 562 |
13.7 (Optional) Polymorphism, Virtual Functions and Dynamic Binding "Under the Hood" | p. 566 |
13.8 Case Study: Payroll System Using Polymorphism and Runtime Type Information with Downcasting, dynamic_cast, typeid and type_info | p. 569 |
13.9 Virtual Destructors | p. 573 |
13.10 Wrap-Up | p. 573 |
14 Templates | p. 579 |
14.1 Introduction | p. 580 |
14.2 Function Templates | p. 580 |
14.3 Overloading Function Templates | p. 583 |
14.4 Class Templates | p. 584 |
14.5 Nontype Parameters and Default Types for Class Templates | p. 590 |
14.6 Wrap-Up | p. 591 |
15 Stream Input/Output | p. 595 |
15.1 Introduction | p. 596 |
15.2 Streams | p. 597 |
15.2.1 Classic Streams vs. Standard Streams | p. 597 |
15.2.2 iostream Library Headers | p. 598 |
15.2.3 Stream Input/Output Classes and Objects | p. 598 |
15.3 Stream Output | p. 601 |
15.3.1 Output of char * Variables | p. 601 |
15.3.2 Character Output Using Member Function put | p. 601 |
15.4 Stream Input | p. 602 |
15.4.1 get and getline Member Functions | p. 602 |
15.4.2 istream Member Functions peek, putback and ignore | p. 605 |
15.4.3 Type-Safe I/O | p. 605 |
15.5 Unformatted I/O Using read, write and gcount | p. 605 |
15.6 Introduction to StreamManipulators | p. 606 |
15.6.1 Integral Stream Base: dec, oct, hex and setbase | p. 607 |
15.6.2 Floating-Point Precision (precision, setprecision) | p. 607 |
15.6.3 Field Width (width, setw) | p. 609 |
15.6.4 User-Defined Output Stream Manipulators | p. 610 |
15.7 Stream Format States and Stream Manipulators | p. 612 |
15.7.1 Trailing Zeros and Decimal Points (showpoint) | p. 612 |
15.7.2 Justification (left, right and internal) | p. 613 |
15.7.3 Padding (fill, setfill) | p. 615 |
15.7.4 Integral Stream Base (dec, oct, hex, showbase) | p. 616 |
15.7.5 Floating-Point Numbers; Scientific and Fixed Notation (scientific, fixed) | p. 617 |
15.7.6 Uppercase/Lowercase Control (uppercase) | p. 618 |
15.7.7 Specifying Boolean Format (boolalpha) | p. 618 |
15.7.8 Setting and Resetting the Format State via Member Function flags | p. 619 |
15.8 Stream Error States | p. 620 |
15.9 Tying an Output Stream to an Input Stream | p. 622 |
15.10 Wrap-Up | p. 623 |
16 Exception Handling: A Deeper Look | p. 632 |
16.1 Introduction | p. 633 |
16.2 Example: Handling an Attempt to Divide by Zero | p. 633 |
16.3 When to Use Exception Handling | p. 639 |
16.4 Rethrowing an Exception | p. 640 |
16.5 Exception Specifications | p. 641 |
16.6 Processing Unexpected Exceptions | p. 642 |
16.7 Stack Unwinding | p. 642 |
16.8 Constructors, Destructors and Exception Handling | p. 644 |
16.9 Exceptions and Inheritance | p. 645 |
16.10 Processing new Failures | p. 645 |
16.11 Class unique_ptr and Dynamic Memory Allocation | p. 648 |
16.12 Standard Library Exception Hierarchy | p. 650 |
16.13 Wrap-Up | p. 652 |
17 File Processing | p. 658 |
17.1 Introduction | p. 659 |
17.2 Files and Streams | p. 659 |
17.3 Creating a Sequential File | p. 660 |
17.4 Reading Data from a Sequential File | p. 664 |
17.5 Updating Sequential Files | p. 669 |
17.6 Random-Access Files | p. 670 |
17.7 Creating a Random-Access File | p. 671 |
17.8 Writing Data Randomly to a Random-Access File | p. 675 |
17.9 Reading from a Random-Access File Sequentially | p. 677 |
17.10 Case Study: A Transaction-Processing Program | p. 679 |
17.11 Object Serialization | p. 686 |
17.12 Wrap-Up | p. 686 |
18 Class string and String Stream Processing | p. 696 |
18.1 Introduction | p. 697 |
18.2 string Assignment and Concatenation | p. 698 |
18.3 Comparing strings | p. 700 |
18.4 Substrings | p. 703 |
18.5 Swapping strings | p. 703 |
18.6 string Characteristics | p. 704 |
18.7 Finding Substrings and Characters in a string | p. 706 |
18.8 Replacing Characters in a string | p. 708 |
18.9 Inserting Characters into a string | p. 710 |
18.10 Conversion to C-Style Pointer-Based char * Strings | p. 711 |
18.11 Iterators | p. 713 |
18.12 String Stream Processing | p. 714 |
18.13 Wrap-Up | p. 717 |
19 Searching and Sorting | p. 724 |
19.1 Introduction | p. 725 |
19.2 Searching Algorithms | p. 725 |
19.2.1 Efficiency of Linear Search | p. 726 |
19.2.2 Binary Search | p. 727 |
19.3 Sorting Algorithms | p. 732 |
19.3.1 Efficiency of Selection Sort | p. 733 |
19.3.2 Efficiency of Insertion Sort | p. 733 |
19.3.3 Merge Sort (A Recursive Implementation) | p. 733 |
19.4 Wrap-Up | p. 740 |
20 Custom Templatized Data Structures | p. 746 |
20.1 Introduction | p. 747 |
20.2 Self-Referential Classes | p. 748 |
20.3 Dynamic Memory Allocation and Data Structures | p. 749 |
20.4 Linked Lists | p. 749 |
20.5 Stacks | p. 764 |
20.6 Queues | p. 768 |
20.7 Trees | p. 772 |
20.8 Wrap-Up | p. 780 |
21 Bits, Characters, C Strings and structs | p. 791 |
21.1 Introduction | p. 792 |
21.2 Structure Definitions | p. 792 |
21.3 typedef | p. 794 |
21.4 Example: Card Shuffling and Dealing Simulation | p. 794 |
21.5 Bitwise Operators | p. 797 |
21.6 Bit Fields | p. 806 |
21.7 Character-Handling Library | p. 810 |
21.8 Pointer-Based String Manipulation Functions | p. 815 |
21.9 Pointer-Based String-Conversion Functions | p. 822 |
21.10 Search Functions of the Pointer-Based String-Handling Library | p. 827 |
21.11 Memory Functions of the Pointer-Based String-Handling Library | p. 831 |
21.12 Wrap-Up | p. 835 |
22 Standard Template Library (STL) | p. 850 |
22.1 Introduction to the Standard Template Library (STL) | p. 851 |
22.2 Introduction to Containers | p. 853 |
22.3 Introduction to Iterators | p. 856 |
22.4 Introduction to Algorithms | p. 861 |
22.5 Sequence Containers | p. 863 |
22.5.1 vector Sequence Container | p. 864 |
22.5.2 list Sequence Container | p. 871 |
22.5.3 deque Sequence Container | p. 875 |
22.6 Associative Containers | p. 877 |
22.6.1 multiset Associative Container | p. 877 |
22.6.2 set Associative Container | p. 880 |
22.6.3 multimap Associative Container | p. 881 |
22.6.4 map Associative Container | p. 883 |
22.7 Container Adapters | p. 885 |
22.7.1 stack Adapter | p. 885 |
22.7.2 queue Adapter | p. 887 |
22.7.3 priority_queue Adapter | p. 888 |
22.8 Algorithms | p. 890 |
22.8.1 fill, fill_n, generate and generate_n | p. 890 |
22.8.2 equal, mismatch and lexicographical_compare | p. 892 |
22.8.3 remove, remove_if, remove_copy and remove_copy_if | p. 895 |
22.8.4 replace, replace_if, replace_copy and replace_copy_if | p. 897 |
22.8.5 Mathematical Algorithms | p. 900 |
22.8.6 Basic Searching and Sorting Algorithms | p. 903 |
22.8.7 swap, iter_swap and swap_ranges | p. 905 |
22.8.8 copy_backward, merge, unique and reverse | p. 906 |
22.8.9 inplace_merge, unique_copy and reverse_copy | p. 909 |
22.8.10 Set Operations | p. 910 |
22.8.11 lower_bound, upper_bound and equal_range | p. 913 |
22.8.12 Heapsort | p. 915 |
22.8.13 min and max | p. 918 |
22.8.14 STL Algorithms Not Covered in This Chapter | p. 919 |
22.9 Class bitset | p. 920 |
22.10 Function Objects | p. 924 |
22.11 Wrap-Up | p. 927 |
23 Boost Libraries, Technical Report 1 and C++0x | p. 936 |
23.1 Introduction | p. 937 |
23.2 Deitel Online C++ and Related Resource Centers | p. 937 |
23.3 Boost Libraries | p. 937 |
23.4 Boost Libraries Overview | p. 938 |
23.5 Regular Expressions with the regex Library | p. 941 |
23.5.1 Regular Expression Example | p. 942 |
23.5.2 Validating User Input with Regular Expressions | p. 944 |
23.5.3 Replacing and Splitting Strings | p. 947 |
23.6 Smart Pointers | p. 950 |
23.6.1 Reference Counted shared_ptr | p. 950 |
23.6.2 weak_ptr: shared_ptr Observer | p. 954 |
23.7 Technical Report 1 | p. 960 |
23.8 C++0x | p. 961 |
23.9 Core Language Changes | p. 962 |
23.10 Wrap-Up | p. 967 |
24 Other Topics | p. 974 |
24.1 Introduction | p. 975 |
24.2 const_cast Operator | p. 975 |
24.3 mutable Class Members | p. 977 |
24.4 namespaces | p. 979 |
24.5 Operator Keywords | p. 982 |
24.6 Pointers to Class Members (.* and -> *) | p. 984 |
24.7 Multiple Inheritance | p. 986 |
24.8 Multiple Inheritance and virtual Base Classes | p. 991 |
24.9 Wrap-Up | p. 996 |
Chapters on the Web | p. 1001 |
A Operator Precedence and Associativity | p. 1002 |
B ASCII Character Set | p. 1004 |
C Fundamental Types | p. 1005 |
D Number Systems | p. 1007 |
D.1 Introduction | p. 1008 |
D.2 Abbreviating Binary Numbers as Octal and Hexadecimal Numbers | p. 1011 |
D.3 Converting Octal and Hexadecimal Numbers to Binary Numbers | p. 1012 |
D.4 Converting from Binary, Octal or Hexadecimal to Decimal | p. 1012 |
D.5 Converting from Decimal to Binary, Octal or Hexadecimal | p. 1013 |
D.6 Negative Binary Numbers: Two's Complement Notation | p. 1015 |
E Preprocessor | p. 1020 |
E.1 Introduction | p. 1021 |
E.2 #include Preprocessor Directive | p. 1021 |
E.3 #define Preprocessor Directive: Symbolic Constants | p. 1022 |
E.4 #define Preprocessor Directive: Macros | p. 1022 |
E.5 Conditional Compilation | p. 1024 |
E.6 #error and #pragma Preprocessor Directives | p. 1025 |
E.7 Operators # and ## | p. 1026 |
E.8 Predefined Symbolic Constants | p. 1026 |
E.9 Assertions | p. 1027 |
E.10 Wrap-Up | p. 1027 |
Appendices on theWeb | p. 1033 |
Index | p. 1035 |
Chapters 25 26 and Appendices F I are PDF documents posted online at the book's | |
Companion Website, which is accessible from www.pearsonhighered.com/deitel. | |
25 ATM Case Study | |
part 1 Object-Oriented Design with the UML | p. 1 |
25.1 Introduction | p. 2 |
25.2 Introduction to Object-Oriented Analysis and Design | p. 2 |
25.3 Examining the ATM Requirements Document | p. 3 |
25.4 Identifying the Classes in the ATM Requirements Document | p. 10 |
25.5 Identifying Class Attributes | p. 17 |
25.6 Identifying Objects' States and Activities | p. 21 |
25.7 Identifying Class Operations | p. 25 |
25.8 Indicating Collaboration Among Objects | p. 32 |
25.9 Wrap-Up | p. 39 |
26 ATM Case Study | |
Part 2 Implementing an Object-Oriented Design | p. 1 |
26.1 Introduction | p. 2 |
26.2 Starting to Program the Classes of the ATM System | p. 2 |
26.3 Incorporating Inheritance into the ATM System | p. 8 |
26.4 ATMCase Study Implementation | p. 15 |
26.4.1 Class ATM | p. 16 |
26.4.2 Class Screen | p. 23 |
26.4.3 Class Keypad | p. 25 |
26.4.4 Class CashDispenser | p. 26 |
26.4.5 Class DepositSlot | p. 28 |
26.4.6 Class Account | p. 29 |
26.4.7 Class BankDatabase | p. 31 |
26.4.8 Class Transaction | p. 35 |
26.4.9 Class BalanceInquiry | p. 37 |
26.4.10 Class Withdrawal | p. 39 |
26.4.11 Class Deposit | p. 44 |
26.4.12 Test Program ATMCaseStudy.cpp | p. 47 |
26.5 Wrap-Up | p. 47 |
F C Legacy Code Topics | p. 1 |
F.1 Introduction | p. 2 |
F.2 Redirecting Input/Output on UNIX/Linux/Mac OS X and Windows Systems | p. 2 |
F.3 Variable-Length Argument Lists | p. 3 |
F.4 Using Command-Line Arguments | p. 5 |
F.5 Notes on Compiling Multiple-Source-File Programs | p. 7 |
F.6 Program Termination with exit and atexit | p. 9 |
F.7 Type Qualifier volatile | p. 10 |
F.8 Suffixes for Integer and Floating-Point Constants | p. 10 |
F.9 Signal Handling | p. 11 |
F.10 Dynamic Memory Allocation with calloc and realloc | p. 13 |
F.11 Unconditional Branch: goto | p. 14 |
F.12 Unions | p. 15 |
F.13 Linkage Specifications | p. 18 |
F.14 Wrap-Up | p. 19 |
G UML 2: Additional Diagram Types | p. 1 |
G.1 Introduction | p. 1 |
G.2 Additional Diagram Types | p. 2 |
H Using the Visual Studio Debugger | p. 1 |
H.1 Introduction | p. 2 |
H.2 Breakpoints and the Continue Command | p. 2 |
H.3 Locals and Watch Windows | p. 8 |
H.4 Controlling Execution Using the Step Into, Step Over, Step Out and Continue Commands | p. 11 |
H.5 Autos Window | p. 13 |
H.6 Wrap-Up | p. 14 |
I Using the GNU C++ Debugger | p. 1 |
I.1 Introduction | p. 2 |
I.2 Breakpoints and the run, stop, continue and print Commands | p. 2 |
I.3 print and set Commands | p. 8 |
I.4 Controlling Execution Using the step, finish and next Commands | p. 10 |
I.5 watch Command | p. 13 |
I.6 Wrap-Up | p. 15 |