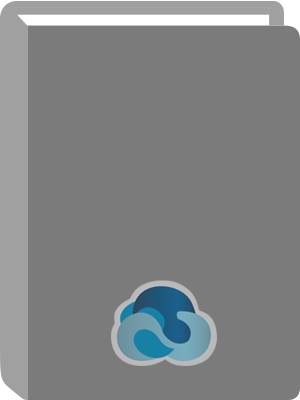
Title:
Oracle 9i JDBC : programming
Personal Author:
Publication Information:
New York : McGraw-Hill, 2002
ISBN:
9780072222548
Available:*
Library | Item Barcode | Call Number | Material Type | Item Category 1 | Status |
---|---|---|---|---|---|
Searching... | 30000010028981 | QA76.9.D3 P743 2002 | Open Access Book | Book | Searching... |
On Order
Summary
Summary
This text covers how to use JDBC programs with the Oracle8i and 9i databases, including details on the Oracle extensions to JDBC. It demonstrates accessing a database from JDBC using examples and introduces JDeveloper, which provides a productive environment for developing Java programs.
Table of Contents
Acknowledgments | p. xv |
Introduction | p. xvii |
Part I Basic JDBC Programming | |
1 Introduction to JDBC | p. 3 |
Software Requirements | p. 4 |
The Oracle Client Software | p. 6 |
Configuration | p. 6 |
Setting the ORACLE_HOME Environment Variable | p. 7 |
Setting the JAVA_HOME Environment Variable | p. 8 |
Setting the PATH Environment Variable | p. 9 |
Setting the CLASSPATH Environment Variable | p. 9 |
Setting the LD_LIBRARY_PATH Environment Variable on Unix or Linux | p. 10 |
Your First JDBC Program | p. 11 |
Example Program: FirstExample.java | p. 11 |
Compiling and Running FirstExample.java | p. 16 |
Oracle JDeveloper | p. 18 |
Creating a New Workspace and Project | p. 18 |
Adding FirstExample.java to the Project | p. 20 |
Adding the Oracle JDBC Library to the Project and Compiling and Running FirstExample.java | p. 23 |
Debugging FirstExample.java | p. 25 |
2 Introduction to Databases and Oracle | p. 29 |
What Is a Relational Database? | p. 30 |
Structured Query Language (SQL) | p. 31 |
SQL *Plus | p. 32 |
Running the store_user.sql Script | p. 32 |
Data Definition Language (DDL) Statements | p. 33 |
Data Manipulation Language (DML) Statements | p. 41 |
Oracle PL/SQL | p. 67 |
Block Structure | p. 68 |
Variables and Types | p. 69 |
Conditional Logic | p. 70 |
Loops | p. 70 |
Cursors | p. 72 |
Procedures | p. 77 |
Functions | p. 80 |
Packages | p. 82 |
3 The Basics of JDBC Programming | p. 85 |
The Oracle JDBC Drivers | p. 86 |
The Thin Driver | p. 86 |
The OCI Driver | p. 87 |
The Server-Side Internal Driver | p. 87 |
The Server-Side Thin Driver | p. 88 |
Importing the JDBC Packages | p. 88 |
Registering the Oracle JDBC Drivers | p. 88 |
Opening a Database Connection | p. 89 |
Connecting to the Database Using the getConnection() Method of the DriverManager Class | p. 89 |
Connecting to the Database Using an Oracle Data Source | p. 92 |
Creating a JDBC Statement Object | p. 95 |
Retrieving Rows from the Database | p. 96 |
Step 1 Create and Populate a ResultSet Object | p. 97 |
Step 2 Read the Column Values from the ResultSet Object | p. 98 |
Step 3 Close the ResultSet Object | p. 101 |
Adding Rows to the Database | p. 102 |
Modifying Rows in the Database | p. 103 |
Deleting Rows from the Database | p. 103 |
Handling Numbers | p. 104 |
Handling Database Null Values | p. 105 |
Controlling Database Transactions | p. 108 |
Performing Data Definition Language Statements | p. 109 |
Handling Exceptions | p. 109 |
Closing Your JDBC Objects | p. 111 |
Example Program: BasicExample1.java | p. 113 |
Prepared SQL Statements | p. 120 |
The Oracle JDBC Extensions | p. 123 |
The oracle.sql Package | p. 124 |
The oracle.jdbc Package | p. 128 |
Example Program: BasicExample3.java | p. 133 |
Part II Advanced JDBC Programming | |
4 Advanced Result Sets | p. 139 |
Scrollable Result Sets | p. 140 |
Navigating a Scrollable Result Set | p. 143 |
Determining the Position in a Scrollable Result Set | p. 145 |
Example Program: AdvResultSetExample1.java | p. 146 |
Updatable Result Sets | p. 149 |
Updating a Row | p. 150 |
Deleting a Row | p. 152 |
Conflicts When Updating and Deleting Rows Using an Updatable Result Set | p. 152 |
Inserting a Row | p. 153 |
Example Program: AdvResultSetExample2.java | p. 154 |
What Database Changes does a Result Set "See"? | p. 157 |
The refreshRow() Method | p. 158 |
When Are Changes Visible? | p. 160 |
Scrollable Sensitive Result Sets | p. 160 |
Example Program: AdvResultSetExample4.java | p. 161 |
Meta Data | p. 164 |
Example Program: MetaDataExample.java | p. 167 |
5 PL/SQL and JDBC | p. 171 |
Calling PL/SQL Procedures | p. 172 |
Calling PL/SQL Functions | p. 175 |
Example Program: PLSQLExample1.java | p. 178 |
Using PL/SQL Packages and REF CURSORs | p. 182 |
6 Database Objects | p. 185 |
Creating Object Types | p. 186 |
Using Object Types to Define Column Objects and Object Tables | p. 188 |
Object References and Object Identifiers | p. 189 |
Performing DML on the products Table | p. 190 |
Inserting Rows into the products Table | p. 190 |
Selecting Rows from the products Table | p. 191 |
Updating a Row in the products Table | p. 192 |
Deleting a Row from the products Table | p. 192 |
Performing DML on the object_products Table | p. 192 |
Inserting Rows into the object_products Table | p. 192 |
Selecting Rows from the object_products Table | p. 193 |
Updating a Row in the object_products Table | p. 194 |
Deleting a Row from the object_products Table | p. 194 |
Performing DML on the object_customers Table | p. 194 |
Inserting Rows into the object_customers Table | p. 194 |
Selecting Rows from the object_customers Table | p. 195 |
Performing DML on the purchases Table | p. 196 |
Inserting a Row into the purchases Table | p. 196 |
Selecting a Row from the purchases Table | p. 197 |
Updating a Row in the purchases Table | p. 197 |
Oracle9i Database Type Inheritance | p. 198 |
NOT INSTANTIABLE Object Types | p. 199 |
Accessing Database Objects Using Weakly Typed Java Objects | p. 200 |
Inserting a Database Object Using a STRUCT | p. 201 |
Selecting Database Objects into a STRUCT | p. 204 |
Updating a Database Object Using a STRUCT | p. 207 |
Deleting an Object | p. 209 |
Example Program: ObjectExample1.java | p. 209 |
Weakly Typed Object References | p. 216 |
Example Program: ObjectExample2.java | p. 220 |
Strongly Typed Interfaces and Custom Classes | p. 226 |
Main Differences Between SQLData and ORAData | p. 227 |
Generating Custom Classes Using JPublisher | p. 227 |
Running JPublisher from the Command Line | p. 228 |
Running JPublisher from JDeveloper | p. 234 |
Using Custom Classes that Implement SQLData | p. 234 |
Type Maps for SQLData Implementations | p. 235 |
Inserting a Database Object Using a Custom Java Object | p. 236 |
Selecting Database Objects into a Custom Java Object | p. 237 |
Updating a Database Object Using a Custom Java Object | p. 238 |
Example Program: ObjectExample3.java | p. 240 |
Using Custom Classes that Implement ORAData | p. 245 |
Generating Custom Classes That Implement ORAData Using JPublisher | p. 245 |
Inserting, Selecting, and Updating Database Objects Using Custom Java Objects | p. 250 |
Example Program: ObjectExample4.java | p. 252 |
Strongly Typed Object References | p. 258 |
7 Collections | p. 265 |
Varrays | p. 267 |
Creating a Varray Type | p. 267 |
Using a Varray Type to Define a Column in a Table | p. 267 |
Populating a Varray with Elements | p. 268 |
Selecting Varray Elements | p. 268 |
Modifying Varray Elements | p. 268 |
Nested Tables | p. 269 |
Creating a Nested Table Type | p. 269 |
Using a Nested Table Type to Define a Column in a Table | p. 269 |
Populating a Nested Table with Elements | p. 270 |
Selecting Nested Table Elements | p. 270 |
Modifying Nested Table Elements | p. 271 |
Oracle9i Multilevel Collection Types | p. 272 |
Accessing Collections Using Weakly Typed Objects | p. 274 |
Inserting a Collection Using an ARRAY | p. 274 |
Selecting Collections into an ARRAY | p. 278 |
Updating a Collection Using an ARRAY | p. 279 |
Example Program: CollectionExample1.java | p. 283 |
Accessing Collections Using Strongly Typed Objects | p. 288 |
Generating the Custom Classes Using JPublisher | p. 289 |
Inserting a Collection Using a Custom Java Object | p. 293 |
Selecting Collections into a Custom Java Object | p. 294 |
Updating a Collection Using a Custom Java Object | p. 296 |
Example Program: CollectionExample3.java | p. 299 |
8 Large Objects | p. 305 |
The Example Files | p. 306 |
Large Objects (LOBs) | p. 307 |
The Example Tables | p. 308 |
The Put, Get, and Stream Methods | p. 309 |
Using the Put Methods to Write to CLOB and BLOB Columns | p. 311 |
Using Streams to Write to CLOB and BLOB Columns | p. 317 |
Storing Pointers to External Files Using BFILE Columns | p. 321 |
Example Program: LobExample1.java | p. 322 |
Using the Get Methods to Read from CLOB and BLOB Columns | p. 328 |
Using Streams to Read from CLOB and BLOB Columns | p. 331 |
Reading External Files Using BFILE Pointers | p. 333 |
Example Program: LobExample2.java | p. 336 |
LONG and LONG RAW Columns | p. 343 |
Writing to LONG and LONG RAW Columns | p. 344 |
Example Program: LongExample1.java | p. 346 |
Reading from LONG and LONG RAW Columns | p. 350 |
9 Advanced Transaction Control | p. 355 |
ACID Transaction Properties | p. 356 |
Transaction Isolation | p. 357 |
A Worked Example Using JDBC | p. 358 |
Example Program: AdvTransExample1.java | p. 362 |
Distributed Transactions | p. 366 |
Example Program: AdvTransExample2.java | p. 367 |
Part III Deploying Java | |
10 Java Stored Procedures and Triggers | p. 373 |
The Oracle JVM Architecture | p. 374 |
Features of Java Stored Programs | p. 376 |
Using the Default Database Connection | p. 377 |
Output | p. 377 |
A Worked Example | p. 379 |
Publishing and Calling Java Methods Using PL/SQL Packages | p. 397 |
Calling Java Stored Programs from PL/SQL Procedures | p. 398 |
Calling Java Stored Programs from Java | p. 399 |
Loading and Publishing Java Stored Programs Using JDeveloper | p. 402 |
Database Triggers | p. 413 |
11 Oracle9iAS Containers for J2EE (OC4J) | p. 421 |
Overview of OC4J | p. 422 |
Installing OC4J | p. 423 |
Starting, Restarting, and Shutting Down OC4J | p. 424 |
Starting OC4J | p. 424 |
Restarting OC4J | p. 424 |
Shutting Down OC4J | p. 425 |
Defining a Data Source | p. 425 |
Servlets | p. 426 |
Deploying the Servlet | p. 429 |
Invoking the Servlet | p. 430 |
JavaServer Pages (JSP) | p. 430 |
Enterprise JavaBeans (EJB) | p. 434 |
An Example Session Bean | p. 435 |
Using the Bean in a Stand-Alone Java Program | p. 447 |
Using the Bean in a Servlet | p. 451 |
Part IV Performance | |
12 Connection Pooling and Caching | p. 457 |
Connection Pooling and Caching Packages | p. 458 |
Connection Pooling with the Thin Driver | p. 459 |
Example Program: ConnectionPoolExample1.java | p. 463 |
Connection Caching | p. 465 |
Creating a Connection Cache | p. 466 |
Requesting, Using, and Closing a Connection Instance | p. 467 |
Closing a Connection Cache | p. 469 |
Example Program: ConnectionCacheExample1.java | p. 469 |
Controlling the Number of PooledConnection Objects | p. 472 |
Example Program: ConnectionCacheExample2.java | p. 474 |
Connection Pooling with the OCI Driver | p. 477 |
Creating an OCI Connection Pool | p. 478 |
Requesting, Using, and Closing a Connection Instance | p. 480 |
Closing the OCI Connection Pool | p. 481 |
Example Program: ConnectionPoolExample2.java | p. 481 |
13 Performance Tuning | p. 485 |
The perf_test Table | p. 486 |
Disabling Auto-Commit Mode | p. 486 |
Example Program: AutoCommitExample.java | p. 487 |
Batching | p. 490 |
Standard Update Batching | p. 491 |
Oracle Update Batching | p. 498 |
Row Prefetching | p. 504 |
Standard Row Prefetching | p. 505 |
Oracle Row Prefetching | p. 510 |
Defining the Types of Result Set Columns | p. 515 |
The defineColumnType() Method | p. 515 |
Defining the Length for a Column | p. 516 |
Structured Objects, Object References, and Array Columns | p. 517 |
Example Program: DefineColumnTypeExample.java | p. 517 |
Statement Caching | p. 519 |
Enabling Statement Caching and Setting the Statement Cache Size | p. 520 |
Types of Statement Caching | p. 521 |
Using Implicit Statement Caching | p. 521 |
Using Explicit Statement Caching | p. 528 |
Tuning SQL Statements | p. 534 |
Use a WHERE Clause to Restrict the Rows | p. 534 |
Add Additional Indexes | p. 534 |
A Final Note | p. 534 |
Part V Appendixes | |
A Oracle and Java Type Mappings | p. 537 |
JDBC 2.0 Type Mappings | p. 538 |
Oracle Extended JDBC Type Mappings | p. 539 |
Java Wrapper Type Mappings | p. 540 |
B Oracle Java Tools Reference | p. 541 |
JPublisher | p. 542 |
loadjava | p. 543 |
dropjava | p. 549 |
ncomp | p. 551 |
deploync | p. 554 |
statusnc | p. 555 |
C Selected JDBC Interface and Class Reference | p. 557 |
Get and Update Methods of the java.sql.ResultSet Interface | p. 558 |
The Get Methods of java.sql.ResultSet | p. 559 |
The Update Methods of java.sql.ResultSet | p. 561 |
Get and Update Methods of the oracle.jdbc.OracleResultSet Interface | p. 563 |
The Get Methods of oracle.jdbc.OracleResultSet | p. 563 |
The Update Methods of oracle.jdbc.OracleResultSet | p. 565 |
Set Methods of the java.sql.PreparedStatement Interface | p. 567 |
Set Methods of the oracle.jdbc.OraclePreparedStatement Interface | p. 569 |
Integer Constants of the java.sql.Types Class | p. 570 |
Integer Constants of the oracle.jdbc.OracleTypes Class | p. 571 |
D JNDI and Data Sources | p. 573 |
Binding a Data Source to JNDI | p. 574 |
Step 1 Create an OracleDataSource Object | p. 575 |
Step 2 Set the Attributes for the OracleDataSource Object | p. 575 |
Step 3 Create a Properties Object | p. 575 |
Step 4 Add the JNDI Properties to the Properties Object | p. 576 |
Step 5 Create a JNDI Context Object | p. 576 |
Step 6 Bind the OracleDataSource Object to JNDI Using the Context Object | p. 576 |
Example Program: JNDIExample1.java | p. 577 |
Looking Up a Data Source Using JNDI | p. 578 |
Step 4 Look Up the Data Source Using JNDI | p. 579 |
Example Program: JNDIExample2.java | p. 579 |
Index | p. 583 |