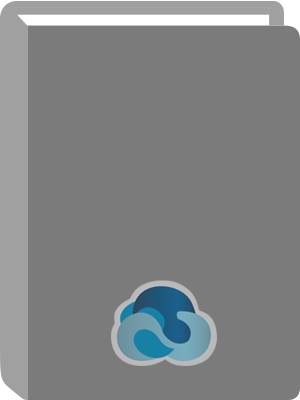
Available:*
Library | Item Barcode | Call Number | Material Type | Item Category 1 | Status |
---|---|---|---|---|---|
Searching... | 30000010053809 | QA76.64 D44 2002 | Open Access Book | Book | Searching... |
Searching... | 30000010053808 | QA76.64 D44 2002 | Open Access Book | Book | Searching... |
On Order
Summary
Summary
The primary focus of Nick DeLilloAEs new book is on object-oriented design (OOD) using the Standard Template Library (STL). The STL provides reusable, reliable components for software design so students donAEt have to be concerned with the correctness and efficiency of the code they design. The author assumes students have prior knowledge of data structures and algorithms, then builds upon this knowledge by introducing the use of the STL. Chapters 1-4 serve as a review of Data Structures and Algorithms including such topics as encapsulation, inheritance, polymorphism, and traditional data structures. In Chapter 5, the transition is made to using the STL to accomplish these same tasks, enabling students to see the benefit of using these predefined tools. The students also are introduced to OOD projects and how the STL is a powerful tool for this type of work. While several texts may cover pieces of these topics, this is the first text that covers them in one comprehensive book."
Table of Contents
Chapter 1 Classes | p. 1 |
Chapter Objectives | p. 1 |
1.1 Introduction | p. 1 |
1.2 Principles of Object-Oriented Design | p. 2 |
1.3 Classes and Objects | p. 7 |
1.4 Constructors and Destructors: An Example | p. 9 |
1.5 Implementation Details | p. 13 |
1.6 Templates | p. 15 |
1.7 An Abstraction for Complex Numbers | p. 23 |
1.8 Suggested Improvements for the Design of the complex Class | p. 30 |
1.9 Exceptions and Exception Handling | p. 33 |
1.10 static Members of a Class | p. 38 |
1.11 Chapter Summary | p. 44 |
Exercises | p. 46 |
Programming Projects | p. 52 |
References | p. 53 |
Chapter 2 Inheritance and Polymorphism | p. 54 |
Chapter Objectives | p. 54 |
2.1 Introduction | p. 54 |
2.2 Inheritance, Base Classes, and Derived Classes | p. 55 |
2.3 public Inheritance | p. 58 |
2.4 protected Members of a Base Class | p. 60 |
2.5 private and protected Inheritance | p. 66 |
2.6 Multiple Inheritance | p. 67 |
2.7 Polymorphism and virtual Functions | p. 71 |
2.8 Pure virtual Functions and Abstract Classes | p. 75 |
2.9 Implications of Inheritance and Polymorphism for Software Engineering | p. 82 |
2.10 Chapter Summary | p. 83 |
Exercises | p. 85 |
Programming Project | p. 98 |
Chapter 3 Search and Sort | p. 100 |
Chapter Objectives | p. 100 |
3.1 Introduction | p. 100 |
3.2 The Concept of an Algorithm | p. 101 |
3.3 Design with Classes and Objects | p. 102 |
3.4 Efficiency Issues: Preliminary Discussion | p. 103 |
3.5 The Principle of Finite Induction | p. 104 |
3.6 Comparing Algorithms: Big-O Notation | p. 105 |
3.7 Search Algorithms for Arrays. Linear (Sequential) Search | p. 106 |
3.8 Analysis of Linear Search | p. 108 |
3.9 Review of Recursive Programming | p. 109 |
3.10 Binary Search | p. 112 |
3.11 Analysis of Binary Search | p. 115 |
3.12 Sorting Algorithms: Selection Sort and Insertion Sort | p. 115 |
3.13 Analysis of Selection Sort and Insertion Sort | p. 118 |
3.14 Quicksort and Recursive Algorithms | p. 119 |
3.15 Analysis of Quicksort | p. 125 |
3.16 Mergesort | p. 126 |
3.17 Analysis of Mergesort | p. 131 |
3.18 Chapter Summary | p. 132 |
Exercises | p. 133 |
Programming Projects | p. 136 |
References | p. 138 |
Chapter 4 Hashing: Prelude to the Standard Template Library | p. 139 |
Chapter Objectives | p. 139 |
4.1 Introduction | p. 139 |
4.2 Hashing as an Efficient Method of Data Storage and Retrieval | p. 140 |
4.3 Choosing an Appropriate Hash Function | p. 143 |
4.4 Strategies for Resolving Hash Collisions | p. 146 |
4.5 Resolving Hash Collisions Using Buckets and Linked Lists | p. 151 |
4.6 Implementation of Hashing Using Class Templates | p. 155 |
4.7 Namespaces | p. 163 |
4.8 Chapter Summary | p. 171 |
Exercises | p. 172 |
Programming Project | p. 175 |
References | p. 176 |
Chapter 5 Overview of the Components of the STL | p. 177 |
Chapter Objectives | p. 177 |
5.1 Historical Introduction | p. 177 |
5.2 Overview of the STL and Its Importance | p. 178 |
5.3 Containers | p. 179 |
5.4 Iterators | p. 181 |
5.5 Description and Classification of Iterators | p. 184 |
5.6 Algorithms | p. 197 |
5.7 Function Objects | p. 202 |
5.8 Adaptors | p. 209 |
5.9 Chapter Summary | p. 214 |
Exercises | p. 216 |
Programming Project | p. 219 |
Chapter 6 Sequence Containers | p. 220 |
Chapter Objectives | p. 220 |
6.1 Introduction | p. 220 |
6.2 The vector Class Template | p. 220 |
6.3 The Constructors for vector: explicit Declarations | p. 222 |
6.4 Description of Other Member Functions of vector | p. 226 |
6.5 An Application of the vector Class | p. 238 |
6.6 An Introduction to Deques | p. 241 |
6.7 An Application of the deque Class: Pseudorandom and Random Numbers | p. 242 |
6.8 An Introduction to STL Lists | p. 246 |
6.9 The Standard Class Template list: Basic Member Functions | p. 247 |
6.10 The Standard Class Template list: More Specialized Member Functions | p. 253 |
6.11 Application of the list Class Template: Sorting Randomly Generated Odd and Even Positive Integers | p. 260 |
6.12 Chapter Summary | p. 264 |
Exercises | p. 265 |
Programming Projects | p. 271 |
References | p. 271 |
Chapter 7 Container Adaptors | p. 272 |
Chapter Objectives | p. 272 |
7.1 Introduction | p. 272 |
7.2 The Stack Abstraction | p. 272 |
7.3 Implementation of Stacks Using the stack Container Adaptor | p. 273 |
7.4 Applications of the Stack ADT | p. 276 |
7.5 The Queue Abstraction | p. 290 |
7.6 Implementation of Queues Using STL's queue Container Adaptor | p. 291 |
7.7 Applications of the Queue ADT | p. 291 |
7.8 The Priority Queue Abstraction | p. 303 |
7.9 Applications of the Priority Queue ADT | p. 305 |
7.10 Chapter Summary | p. 312 |
Exercises | p. 314 |
Programming Projects | p. 316 |
Chapter 8 Generic Algorithms | p. 317 |
Chapter Objectives | p. 317 |
8.1 Introduction | p. 317 |
8.2 A General Overview of the Generic Algorithms in the Standard Library | p. 318 |
8.3 Nonmutating Sequence Algorithms | p. 319 |
8.4 Mutating Sequence Algorithms | p. 333 |
8.5 Sorting-Related Algorithms | p. 348 |
8.6 Generalized Numeric Algorithms | p. 365 |
8.7 Chapter Summary | p. 369 |
Exercises | p. 370 |
Programming Project | p. 374 |
References | p. 375 |
Chapter 9 Sorted Associative Containers | p. 376 |
Chapter Objectives | p. 376 |
9.1 Introduction | p. 376 |
9.2 Sets and Multisets | p. 377 |
9.3 Set Operations | p. 388 |
9.4 Implementation Details: Binary Search Trees and Red-Black Trees | p. 394 |
9.5 Maps and Multimaps | p. 403 |
9.6 Applications | p. 409 |
9.7 Chapter Summary | p. 420 |
Exercises | p. 421 |
Programming Projects | p. 423 |
Reference | p. 423 |
Appendix A Local Area Network Simulator | p. 424 |
Glossary | p. 441 |
Index | p. 455 |